2.3: Input, Variables and Arithmetic Operations
- Page ID
- 366492
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\(\newcommand{\avec}{\mathbf a}\) \(\newcommand{\bvec}{\mathbf b}\) \(\newcommand{\cvec}{\mathbf c}\) \(\newcommand{\dvec}{\mathbf d}\) \(\newcommand{\dtil}{\widetilde{\mathbf d}}\) \(\newcommand{\evec}{\mathbf e}\) \(\newcommand{\fvec}{\mathbf f}\) \(\newcommand{\nvec}{\mathbf n}\) \(\newcommand{\pvec}{\mathbf p}\) \(\newcommand{\qvec}{\mathbf q}\) \(\newcommand{\svec}{\mathbf s}\) \(\newcommand{\tvec}{\mathbf t}\) \(\newcommand{\uvec}{\mathbf u}\) \(\newcommand{\vvec}{\mathbf v}\) \(\newcommand{\wvec}{\mathbf w}\) \(\newcommand{\xvec}{\mathbf x}\) \(\newcommand{\yvec}{\mathbf y}\) \(\newcommand{\zvec}{\mathbf z}\) \(\newcommand{\rvec}{\mathbf r}\) \(\newcommand{\mvec}{\mathbf m}\) \(\newcommand{\zerovec}{\mathbf 0}\) \(\newcommand{\onevec}{\mathbf 1}\) \(\newcommand{\real}{\mathbb R}\) \(\newcommand{\twovec}[2]{\left[\begin{array}{r}#1 \\ #2 \end{array}\right]}\) \(\newcommand{\ctwovec}[2]{\left[\begin{array}{c}#1 \\ #2 \end{array}\right]}\) \(\newcommand{\threevec}[3]{\left[\begin{array}{r}#1 \\ #2 \\ #3 \end{array}\right]}\) \(\newcommand{\cthreevec}[3]{\left[\begin{array}{c}#1 \\ #2 \\ #3 \end{array}\right]}\) \(\newcommand{\fourvec}[4]{\left[\begin{array}{r}#1 \\ #2 \\ #3 \\ #4 \end{array}\right]}\) \(\newcommand{\cfourvec}[4]{\left[\begin{array}{c}#1 \\ #2 \\ #3 \\ #4 \end{array}\right]}\) \(\newcommand{\fivevec}[5]{\left[\begin{array}{r}#1 \\ #2 \\ #3 \\ #4 \\ #5 \\ \end{array}\right]}\) \(\newcommand{\cfivevec}[5]{\left[\begin{array}{c}#1 \\ #2 \\ #3 \\ #4 \\ #5 \\ \end{array}\right]}\) \(\newcommand{\mattwo}[4]{\left[\begin{array}{rr}#1 \amp #2 \\ #3 \amp #4 \\ \end{array}\right]}\) \(\newcommand{\laspan}[1]{\text{Span}\{#1\}}\) \(\newcommand{\bcal}{\cal B}\) \(\newcommand{\ccal}{\cal C}\) \(\newcommand{\scal}{\cal S}\) \(\newcommand{\wcal}{\cal W}\) \(\newcommand{\ecal}{\cal E}\) \(\newcommand{\coords}[2]{\left\{#1\right\}_{#2}}\) \(\newcommand{\gray}[1]{\color{gray}{#1}}\) \(\newcommand{\lgray}[1]{\color{lightgray}{#1}}\) \(\newcommand{\rank}{\operatorname{rank}}\) \(\newcommand{\row}{\text{Row}}\) \(\newcommand{\col}{\text{Col}}\) \(\renewcommand{\row}{\text{Row}}\) \(\newcommand{\nul}{\text{Nul}}\) \(\newcommand{\var}{\text{Var}}\) \(\newcommand{\corr}{\text{corr}}\) \(\newcommand{\len}[1]{\left|#1\right|}\) \(\newcommand{\bbar}{\overline{\bvec}}\) \(\newcommand{\bhat}{\widehat{\bvec}}\) \(\newcommand{\bperp}{\bvec^\perp}\) \(\newcommand{\xhat}{\widehat{\xvec}}\) \(\newcommand{\vhat}{\widehat{\vvec}}\) \(\newcommand{\uhat}{\widehat{\uvec}}\) \(\newcommand{\what}{\widehat{\wvec}}\) \(\newcommand{\Sighat}{\widehat{\Sigma}}\) \(\newcommand{\lt}{<}\) \(\newcommand{\gt}{>}\) \(\newcommand{\amp}{&}\) \(\definecolor{fillinmathshade}{gray}{0.9}\)Content:
- builtin functions (input(), print() and type() functions)
- import package from PyPi
- library function from package
- variables (float, string and integer)
- arithmetic operations on both numbers and strings (concatenation)
- acquire data from API
Process:
- Write a program to calculate the mass solute needed to make user defined volume and concentration for a given solute
- 3 inputs are user entered values
- 1 input is called from a web API
Prior Knowledge
- variable types
- arithmetic operations
Further reading
Note: this activity requires you to use an IDE. In this class we will be using the Thonny IDE, which is the default IDE on the Raspberry Pi, but any IDE will work.
Create a folder called chem4399 or chem5399 on your personal computer, and in this create a subfolder called "Python files". In the Python files folder create an additional subfolder called "py03", and in here you will store the files associated with this activity. Remember, you overwrite a file if you change it and then run it on Thonny, so either comment out code you change or save as a new file before running.
Input and Print Functions
In lecture we discussed three "types" of functions:
- "Builtin Functions" - those that come with the standard installation of python
- "Library Functions" - those that can be added by installing a package
- "User Defined Functions" - those that you can make
The input and print functions are of the first type. All functions in python are followed by a parenthesis, which relates to the arguments they can operate on.
input()
In this activity we will use the input() function to input the name, atomic number and atomic mass of an element and assign each to a variable name. We will then use the print() function to print to the shell the values we assign to each variable. After this we will investigate the kind of data each variable represents.
- Open Thonny
- Click File/New
- Click Save, move to the folder py03 and name py03_activty1
- Under the View menu make sure the shell and variables options are checked
- Input the following code in the editor (so they are on line 1 & 2 of the editor)
#1 #This script will investigate inputting data to a variable element_name=input("input the name of an element: ")
In the above code you are using the assignment operator to assign the value you input to the variable "element_name". Run the above script by typing "F5" or hitting the green arrow on the Thonny menu.
Exercise \(\PageIndex{1}\)
In the "variables" window you see the name in quotation marks . What kind of data is the variable element_name
- Answer
-
It is a string because it is in quotation marks.
- Highlight the text and copy the text =input("input the name of an element: ").
- In the next line type atomic_number and paste copied script,
- In the next line type atomic_weight and paste the copied script
- Now change the word "name" to "atomic number" and "atomic weight"
your code should look like this
#2 #This script will investigate inputting data to a variable element_name = input("input the name of an element: ") atomic_number = input("input the atomic number of an element: ") atomic_weight = input("input the atomic weight of an element: ")
Exercise \(\PageIndex{2}\)
What kind of data is the variables element_name, atomic_number and atomic_weight?
- Answer
-
They are all string as when you look at the variables window, they are all in quotation marks.
Caution
The input function inverts everything as a string, including numbers. These must be converted to either floating decimal or integer data types if you wish to perform arithmetic operations on them.
Python allows you to change the data type of a variable. In the next script we will assign the atomic number to an integer and the atomic weight to a floating decimal.
int() and float() functions
- Now convert the variables atomic_number and atomic_weight to integer and floating decimals using the int() and float() functions.
note, (figure \(\PageIndex{1}\)) shows how autocomplete can assist
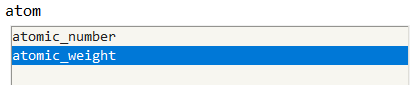
Your code should look like this
#3 #This script will investigate inputting data to a variable element_name = input("input the name of an element: ") atomic_number = input("input the atomic number of an element: ") atomic_weight = input("input the atomic weight of an element: ") atomic_number=int(atomic_number) atomic_weight = float(atomic_weight)
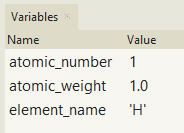
You can use the int() and float() functions when you assign the variables. Here the input() function is nested inside of the int() or float()function
#4 #This script will investigate inputing data to a variable element_name = input("input the name of an element: ") atomic_number = int(input("input the atomic number of an element: ")) atomic_weight = float(input("input the atomic weight of an element: "))
You can even input more than one variable of more than one type in a single line
#5 #This script will investigate inputting data to a variable element_name,atomic_number,atomic_weight = input("Enter element name: "), int(input("Enter atomic_number: ")),float(input("Enter atomic_weight"))
It is best practice not to have more than 80 characters on a line, and so everything to the right can be put into parenthesis and wrapped around multiple lines of code
#6 #This script will investigate inputting data to a variable element_name,atomic_number,atomic_weight = (input("Enter element name: "), int(input("Enter atomic_number: ")),float(input("Enter atomic_weight")))
print()
The print function (print()) is an output that prints the code to the shell. The print function can call variables and embed them into a string.
#7 #This script will investigate inputing data to a variable and printing the value to the shell #the following code inputs three variable values element_name,atomic_number,atomic_weight = (input("Enter element name: "), int(input("Enter atomic_number: ")),float(input("Enter atomic_weight"))) #The following code prints the value that were inputted. print("the element name is ",element_name) print("the atomic number is ",atomic_number) print("the atomic weight is ",atomic_weight)
We can even put the output in a sentence
#8 #This script will investigate inputing data to a variable and printing the value to the shell #the following code inputs three variable values element_name,atomic_number,atomic_weight = (input("Enter element name: "), int(input("Enter atomic_number: ")),float(input("Enter atomic_weight"))) #The following code prints the value that were inputted in a sentence. print("the element",element_name, "has an atomic number of",atomic_number, "and an atomic weight of",atomic_weight,"g/mol")
type()
The type function will tell you the type of an object
Try the following in Thonny (Be sure you ran the code which inputs data for the variables)
#9 #This script will investigate inputing data to a variable and printing the value to the shell #the following code inputs three variable values element_name,atomic_number,atomic_weight = (input("Enter element name: "), int(input("Enter atomic_number: ")),float(input("Enter atomic_weight"))) #The following code prints the value that were inputted in a sentence. # print("The element",element_name, "has an atomic number of",atomic_number, # "and an atomic weight of",atomic_weight,"g/mol") print("hydrogen is of type ", type(element_name)) print("atomic_number is of type ",type(atomic_number)) print("atomic_weight is of type ",type(atomic_weight)) print("The print function is of type ",type(print)) print("The input function is of type ",type(input))
Arithmetic Operations
The following symbols are used for arithmetic operations:
Operation | Addition | Subtraction | Multiplication | Exponentiation | Division | Floor Division | Modulus |
Symbol | + | - | * | ** | / | // | % |
Let's input two numbers and add them
#10 num1=input("Enter a number") num2=input("Enter another number") sum_nums=num1+num2 print("This says the sum is",sum_nums,"which makes no sense")
So in order to do arithmetic operations we first need to convert the strings to either integers or floating decmial data types.
Floor Division and the modulus at first will often confuse students, so let's refresh your elementary school long division, and note that long division gives the quotient and the modulus gives the remainder.
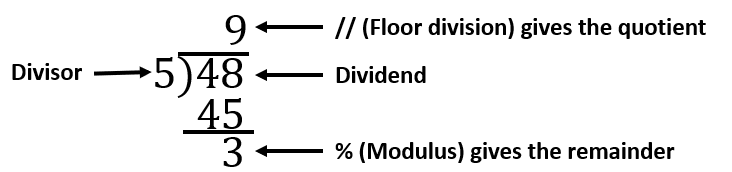
Note, this is commonly written as \(dividend \div divisor \; or\; \frac{dividend}{divisor}\)
#11 THE FOLLOWING CODE DOES NOT WORK: Paste it into Thonny and fix it #following code let's you explore division, floor division and the modulus dividend = input("Enter a whole number dividend: ") divisor = input("Enter a whole number divisor : ") division = dividend/divisor floor_division = dividend//divisor modulus = dividend%divisor print(("the result of division is", division,"which can be expressed in terms of the quotient", floor_division, "and the remainder",modulus))
Concatenation
Merriam-Webster's dictionary defines concatenate to mean "linked together". In code box #10 you did not add your two numbers but you concatenated them. So 1+1=2 if it is an integer, but 1+1= 11 if they are strings. Concatenation can be very useful for obtaining information off the web. In this example we are going to use concatenation with the Pubchem PUG REST API (Power User Gateway Representational State Transfer Application Program Interface) to obtain the molar mass of a chemical. We are going to do this for the common name, but with more effort we could also do this for synonyms. An API is like a GUI (Graphical User Interface) in that the API allows interaction between two computers while the GUI allows a person to interact with a computer. When you navigate to a webpage the web address in the hyperlink is a URL (Universal Resource Locater), while you can also address items within a database by a URI (Universal Resource Identifier). The W3C (World Wide Web Consortium) set forth a RDF (Resource Descriptive Framework) that involves an RDF triple (subject, predicate, object) relationship and we will take advantage of that to obtain the molar mass by concatenating a string into a URI. OK, it looks complicated, but it is simple. The subject is a molecule (which we will input and assign to a variable), the predicate is a property of a molecule (its molar mass, but we could seek other properties) and the object is the value of the molar mass for the molecule, which we will assign to a new variable and be able to use in a calculation.
First, place the following link into a new tab of your browser:
pubchem.ncbi.nlm.nih.gov/rest/pug/compound/name/aspirin/property/MolecularWeight/txt
This should return 180.16, which is the molar mass of aspirin as found in PubChem's compound record for aspirin. If you change the word aspirin in the above link to another molecule, like caffeine, it will give you the molar mass of caffeine. Note, compound words like hydrochloric acid need a dash (hydrochloric-acid).
#12 compound_name = "aspirin" uri = ("https://pubchem.ncbi.nlm.nih.gov/rest/pug/compound/name/" + compound_name +"/property/MolecularWeight/txt") print(uri)
The above script gives you the uri for the value but you wish to assign it to a variable. For this we are going to need to install the request package from the PyPi package index. On the Thonny menu go to Tools/Manage Packages and type in requests and then Search on PyPi. Figure \(\PageIndex{4}\) shows it is already installed on this version of python, but it is probably not installed on yours.
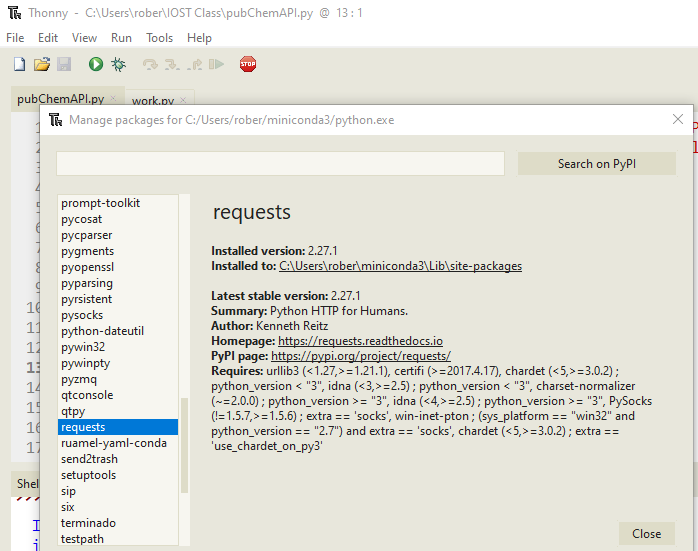
The requests package will give us new functions and classes that we can use to assign the molar mass to a variable.
#13 #This code returns the moles in a sample of a chemical compound using PubChem #obtain the molar mass. We use the requests packet that must be installed in Python import requests compound_name = input("Input compound's name: ") cid = compound_name uri = ("https://pubchem.ncbi.nlm.nih.gov/rest/pug/compound/name/" +str(compound_name) +"/property/MolecularWeight/txt") res = requests.get(uri) molar_mass=float(res.text.rstrip()) mass = float(input("input mass of sample in units of g: ")) moles = mass/molar_mass print("{0} has a molar mass of {1} and {2}g has {3:.4f} moles. " .format(compound_name, molar_mass, mass, moles)) print(cid,"has a molar mass of",molar_mass,"and",mass,"g of",cid,"has",moles,"moles.")
Next week we will look into how to format the print statement, but for now we have shown that there is another way to input data into a program, and that is through a web API. When we start streaming IOT data we will be interacting with other types of web APIs.
String multiplication
You can also multiply strings
#14 spacer=20*" *! " print(spacer)
Assignment:
Write a Python program called Py_03_Molarity_Calculator, which tells you how many grams of a solute you need to make X ml of Y Molar solutions. There are four inputs to this program as described in the following flow chart
You need to make extensive use of comments in your code and the first few lines need to identify who you are, which assignment this is, and what it does.
#15 #Student Name #Py_0X Mole_Calculator '''This code returns the moles in a sample of a chemical compound using PubChem to obtain the molar mass. We use the requests packet that must be installed in Python'''
Note you can either use # to comment out a line, or three quotation marks to enclose a comment. In some IDEs the three quotation marks shows up in the help menu.
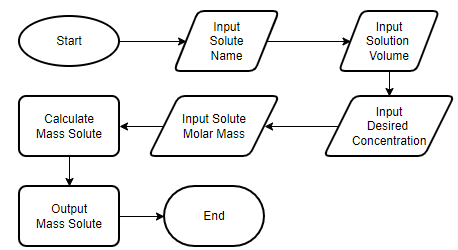
NOTE: Undergrads may either input the molar mass as a typed in variable, or through the PubChem REST API. Grad students need to use the Pubchem REST API. In fact it is strongly encouraged that everyone use the API, as that gives you experience with installing a package in python. The following image is what you should see in the command line

Upload a running version of the program to your Google Drive folder for grading.